Exam 1 Review
[1]
[2]
[3]
[4]
[5]
[6]
[7]
Problem X1r.1.
Suppose we have the following HTML form.
<form method="post" action="grade.php">
<input type="text" name="data" />
<input type="submit" value="Submit" />
</form>
Complete the below file
grade.php so that it outputs
You received an A
when the value typed
in the text field is at least 90;
You received a B
when the text field is at least 80; and
You did somewhat poorly
otherwise.
otherwise.
<?php import_request_variables("pg", "form_"); ?>
<html>
<head><title>Grade Result</title></head>
<body>
<?php
if($form_data >= 90) {
echo "<p>You received an A.</p>";
} elseif($form_data >= 80) {
echo "<p>You received a B.</p>";
} else {
echo "<p>You did somewhat poorly.</p>";
}
?>
</body>
</html>
Problem X1r.2.
Suppose we have the following HTML form, which
contains a blank where a user can type a name.
<form method="post" action="hello.php">
<input type="text" name="name" />
<input type="submit" value="Enter" />
</form>
Complete the
hello.php file below so that when the
user submits the form with a blank name, the response reads
``Please enter a name.'' But when the name isn't blank, the response
should read ``Hello,
name.''
(Here
name stands for whatever the user typed in the
form's blank.)
<?php import_request_variables("pg", "form_"); ?>
<html>
<head><title>Greetings</title></head>
<body>
<?php
if($form_name == "") {
echo "<p>Please enter a name.</p>";
} else {
echo "<p>Hello, ", $form_name, ".</p>";
}
?>
</body>
</html>
Problem X1r.3.
Recall the Lempel-Ziv-Welch data compression algorithm.
1. Initialize table.
2. cur ← first character of file.
3. K ← next character of file.
4. Is cur + K in table?
|
Yes: |
cur ← cur + K.
|
|
No: |
Add cur + K to table.
Output code for cur.
cur ← K
|
6. If characters remain in file, repeat from step 3.
7. Output code for cur.
Suppose the table is initialized to have A mapped to 0, B mapped
to 1, and C mapped to 2, and we're told to compress the string
ABABCABC. What is the resulting code sequence? Show your
intermediate work.
The code sequence is 0,1,3,2,5, as shown by the following
table.
table |
cur |
K |
output |
A:0, B:1, C:2 |
A |
B |
0 |
A:0, B:1, C:2, AB:3 |
B |
A |
1 |
A:0, B:1, C:2, AB:3, BA:4 |
A |
B |
|
A:0, B:1, C:2, AB:3, BA:4 |
AB |
C |
3 |
A:0, B:1, C:2, AB:3, BA:4, ABC:5 |
C |
A |
2 |
A:0, B:1, C:2, AB:3, BA:4, ABC:5, CA:6 |
A |
B |
|
A:0, B:1, C:2, AB:3, BA:4, ABC:5, CA:6 |
AB |
C |
|
A:0, B:1, C:2, AB:3, BA:4, ABC:5, CA:6 |
ABC |
|
5 |
Problem X1r.4.
Recall the following Lempel-Ziv-Welch decompression algorithm.
1. Initialize table.
2. k ← first code in file.
3. Output string for k.
4. old ← k.
5. k ← next code in file.
6. Does code k exist in table?
|
Yes: |
Output string for k.
s ← string for old + first character
of string for k.
Add s to table.
|
|
No: |
s ← string for old + first character
of string for old.
Output s.
Add s to table.
|
7. If codes remain in file, repeat from step 4.
Suppose the table is initialized to have A mapped to 0, B mapped
to 1, and C mapped to 2, and we're told to decompress the code
sequence 0,2,4,3,5. What is the original text? Show your
intermediate work.
table |
old |
k |
output |
A:0, B:1, C:2 |
— |
0 |
A |
A:0, B:1, C:2 |
0 |
2 |
C |
A:0, B:1, C:2, AC:3 |
2 |
4 |
CC |
A:0, B:1, C:2, AC:3, CC:4 |
4 |
3 |
AC |
A:0, B:1, C:2, AC:3, CC:4, CCA:5 |
3 |
5 |
CCA |
Thus the original, uncompressed text is
ACCCACCCA
Problem X1r.5.
Explain the fundamentals of how GIF image compression works.
The image is first reduced to 256 colors, so that each pixel can
be represented using a one-byte value; then Lempel-Ziv-Welch
compression is applied to the sequence of bytes resulting in
reading pixels in row-by-row order.
Problem X1r.6.
What is meant by a
lossy image representation format?
Give an example of lossiness in image formats, and explain why lossy
formats are useful.
If there are images which when saved in the format and then
reopened do not match the original image exactly, then the image format
is lossy. An example is the JPEG format, where the color
information for three-quarters of the pixels is removed.
Lossy formats can often result in dramatically reduced image
sizes without any loss that is readily apparent to the human
eye.
Problem X1r.7.
Consider the following photo of a hummingbird.
We can take this photo, save it in the PNG, JPEG, or GIF
formats, and then blow up the region outlined in red. We get the
following three.
Which is based on a GIF image, which a PNG image, and which a
JPEG image? Explain how you can tell.
JPEG. The colors are somewhat muddier due to the subsampling
of the Cb and Cr components. Also, you can see where the image
has been split into 8×8 blocks, as diagrammed below.
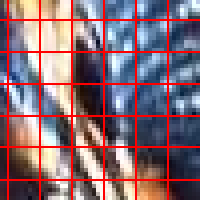
GIF. The reduction to just 256 colors is apparent, most
particularly in the brown region at the lower right (nearest the
bird's beak), where you can see more pixelation than in the other
two photos.
PNG. This image does not have the same evident flaws common to
GIFs or JPEGs, so it must be a PNG. [PNG is completely lossless,
so there aren't any imperfections to look for.]
[Incidentally, the JPEG is 29 kilobytes, the GIF 192 KB, and the
PNG 472 KB. If we reduce the PNG to 256 colors like the GIF, it
requires 168 KB.]